Python正則表達式使用手冊
2024-06-04 加入收藏
正則在處理字符串的領域是無可非議的強大!
正因過于強大,導致正則的使用門檻也不低。
我總結一些常見的匹配方式并結合Python代碼例子,希望對大家使用/使用正則的時候有所幫助!
字符匹配
字符匹配:匹配指定的字符或字符集合,例如「單個字符」[a-z]
、「數字字符」\d
等。
import re
# 匹配單個字符
pattern = r"b[aeiou]t"
string = "bat, bet, bit, bot, but"
result = re.findall(pattern, string)
print(result) # ['bat', 'bet', 'bit', 'bot', 'but']
# 匹配數字字符
pattern = r"\d+"
string = "123 456 789"
result = re.findall(pattern, string)
print(result) # ['123', '456', '789']
位置匹配
位置匹配:匹配字符串的位置,例如「行首」、「行尾」、「單詞邊界」等。
import re
# 匹配行首
pattern = r"^The"
string = "The quick brown fox\nThe lazy dog"
result = re.findall(pattern, string, re.MULTILINE)
print(result) # ['The', 'The']
# 匹配單詞邊界
pattern = r"\bfox\b"
string = "The quick brown fox\njumps over the lazy dog"
result = re.findall(pattern, string)
print(result) # ['fox']
重復匹配
重復匹配:匹配重復出現的字符或字符集合,例如「重復次數」、「重復范圍」等。
import re
# 匹配重復次數
pattern = r"a{3}"
string = "aaa abc aa a"
result = re.findall(pattern, string)
print(result) # ['aaa']
# 匹配重復范圍
pattern = r"\d{2,3}"
string = "12 123 1234 12345"
result = re.findall(pattern, string)
print(result) # ['12', '123', '123', '345']
分支匹配
分支匹配:匹配多個可選項,例如「選項1」或「選項2」。
import re
# 匹配多個可選項
pattern = r"cat|dog"
string = "The quick brown fox jumps over the lazy dog"
result = re.findall(pattern, string)
print(result) # ['dog']
# 匹配多個可選項(忽略大小寫)
pattern = r"cat|dog"
string = "The quick brown Fox jumps over the lazy Dog"
result = re.findall(pattern, string, re.IGNORECASE)
print(result) # ['Fox', 'Dog']
分組匹配
分組匹配:匹配特定的字符或字符集合,并將其標記為子表達式,例如「提取子字符串」。
import re
# 提取子字符串
pattern = r"(\d{4})-(\d{2})-(\d{2})"
string = "2022-03-02 is a good day"
result = re.findall(pattern, string)
print(result) # [('2022', '03', '02')]
后向引用匹配
后向引用匹配:匹配之前已經匹配的子表達式,例如查找重復單詞。
import re
# 提取子字符串
pattern = r"(\d{4})-(\d{2})-(\d{2})"
string = "2022-03-02 is a good day"
result = re.findall(pattern, string)
print(result) # [('2022', '03', '02')]
貪婪匹配和懶惰匹配
貪婪匹配和非貪婪匹配:貪婪匹配是指匹配「盡可能多」的字符,懶惰匹配是指匹配「盡可能少」的字符。
import re
# 貪婪匹配
pattern = r"<.*>"
string = "<a>hello</a><b>world</b>"
result = re.findall(pattern, string)
print(result) # ['<a>hello</a><b>world</b>']
# 非貪婪匹配
pattern = r"<.*?>"
string = "<a>hello</a><b>world</b>"
result = re.findall(pattern, string)
print(result) # ['<a>', '</a>', '<b>', '</b>']
零寬度斷言匹配
零寬度斷言匹配:零斷言可以匹配一個位置,而不是匹配一個字符。
零斷言用于在匹配字符串時,指定匹配的位置前或后必須滿足某些條件,從而實現更加精確的匹配。
在正則表達式中,有四種常用的零斷言:
正向零斷言:匹配滿足正則表達式的字符后面的位置,但不包括這些字符。
import re
# 正向零斷言,匹配hello后面是world的位置
pattern = r"hello(?=world)"
string = "hellopythonhelloworld"
result = re.findall(pattern, string)
print(result) # ['hello']
反向零斷言:匹配不滿足正則表達式的字符后面的位置,但不包括這些字符。
import re
# 反向零斷言,匹配hello后面不是world的位置
pattern = r"hello(?!world)"
string = "hellopythonhelloworld"
result = re.findall(pattern, string)
print(result) # ['hello']
正向零寬度斷言:匹配滿足正則表達式的字符前面的位置,但不包括這些字符。
import re
# 正向零寬度斷言,匹配hello前面是python的位置
pattern = r"(?<=python)hello"
string = "pythonhellopythonworld"
result = re.findall(pattern, string)
print(result) # ['hello']
反向零寬度斷言:匹配不滿足正則表達式的字符前面的位置,但不包括這些字符。
import re
# 反向零寬度斷言,匹配hello前面不是python的位置
pattern = r"(?<!python)hello"
string = "pythonhellopythonworld"
result = re.findall(pattern, string)
print(result) # []
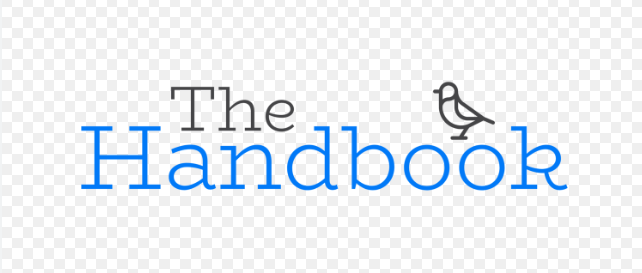
?如果你想加速Python學習,獲得專業的指導,30天學會一門技能!
歡迎參加麥叔Python實戰訓練營,「入門營」,「爬蟲營」,「辦公自動化營」同步開放。
詳情點這里:麥叔Python訓練營
?
如果你希望我更新某個特定小知識,歡迎給我留言。